A variable is a place to store a value in computer memory. You could think of a variable as a cardboard box. You can put an object inside the box, shove the box into a closet, then eventually dig it out again and retrieve the item. Usually, people put a label or other kind of marker on the outside of the box to remember what’s inside.
Variables work the same way: each variable has a name and a value. The name is like the label on a box – a shorthand reminder of what the variable ‘contains’. The value can be any piece of information your PlotDevice script needs to remember: an important number, a piece of text, a list of colors, and so on.
Each script has its own memory storage for variables – a private warehouse of boxed-up values and labels. The specific value of a variable is therefore only known inside the script where it is used, not in other scripts.
Using Variables
You store things in variables when you plan to reuse them. If you’re going to draw a dozen rectangles where each one is a hundred pixels wide, it’s a good idea to declare a width variable that stores the number 100. You can then the rectangles look at what’s inside the width variable (rather than typing the same magic number in every call to the rect() command).
This way, when you change your mind about the rectangles’ width, you only need to change the contents of the variable, since each rectangle is looking there for its width. This is an example of indirection, a key concept in computer science.
Declaring variables
You can create a new variable at any point in your script and give it any name you like, though you should try to stay away from names that PlotDevice has already claimed (like rect, or for). It’s best to pick a name that tells you something about the kind of information the variable is storing.
Try to come up with short, descriptive names. No one is happy about having to type theWidthOfAllTheRectangles
dozens of times; especially when a simple name like width
says enough.
Once you’ve chosen a name you can ‘assign’ any value you like to it with the ‘=
’ operator. You can then use the variable as a stand-in for the value when calling commands:
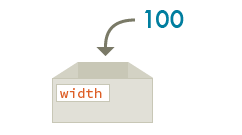
When you declare a new variable, PlotDevice sets aside a metaphorical storage box with a label to remind itself which variable ‘contains’ the new value.
width = 100 rect(10, 10, width, 30)
Changing variables
You can change the value of a variable over the course of a script. When this happens, the old value is thrown away and the variable now contains the new value.
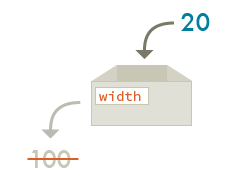
The first rectangle is drawn when the width
value is 100. We then change width
to 20 and draw two more rectangles (which use the newly-set value).
width = 100 rect(10, 10, width, 30 ) width = 20 rect(120, 10, width, 30) rect(330, 10, width, 30)
Calculations with variables
When you store numbers in variables you can perform calculations as though the variable were the actual number. To perform basic ‘calculator arithmetic’, you can use the +
, -
, *
, and /
operators. You can also assign a new value that’s based on the variable’s current value by using one of the augmented assignment operators:
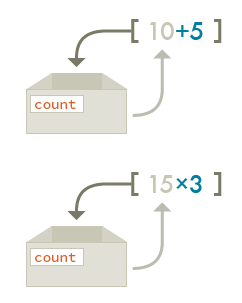
count = 10 print("half of", count, "is", count/2) >>> half of 10 is 5 count += 5 # equivalent to: count = count+5 print(count) >>> 15 count *= 3 # equivalent to: count = count*3 print(count) >>> 45
This comes up quite frequently when laying out sequential blocks of text since the lines’ positions aren’t truly independent. Ideally, paragraph text should go neatly below the title (and when the title font size changes the paragraph text should move along to match it).
Another way of saying this is that the paragraph’s vertical position should be calculated relative to the title’s vertical
position. In this example we use the y
variable to position the title, then update its value to reflect the title’s measured height:
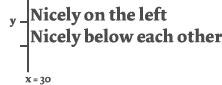
x = 30 y = 30 quote = "Nicely on the left" text(quote, x, y) y += measure(quote).height quote = "Nicely below each other" text(quote, x, y)
Predefined Variables
PlotDevice has a set of predefined variables that contain information about the state of your script. These variables can only be looked at, not modified.
WIDTH
: the width of the drawing areaHEIGHT
: the height of the drawing areaPAGENUM
: the current page in a multi-page export.FRAME
: the current frame in an animation.
Other variables can be used to create interactive animations:
MOUSEX
: the horizontal location of the mouse cursorMOUSEY
: the vertical location of the mouse cursormousedown
: is True when the mouse button is pressed, False otherwisekeydown
: is True when a key is being pressed, False otherwisekey
: the last key pressedkeycode
: the integer keycode of the last key pressedKEY_UP
,KEY_DOWN_
KEY_LEFT
,KEY_RIGHT
,KEY_BACKSPACE
contain the keycodes for the arrow keys and the backspace key.
You can see the full list of global variables PlotDevice maintains for you in the reference section on Constants.
The Variables Panel
The variables panel displays script variables visually as sliders, fields, or check boxes. When you drag a variable’s slider, its value changes on the fly and will affect the output in the drawing area.
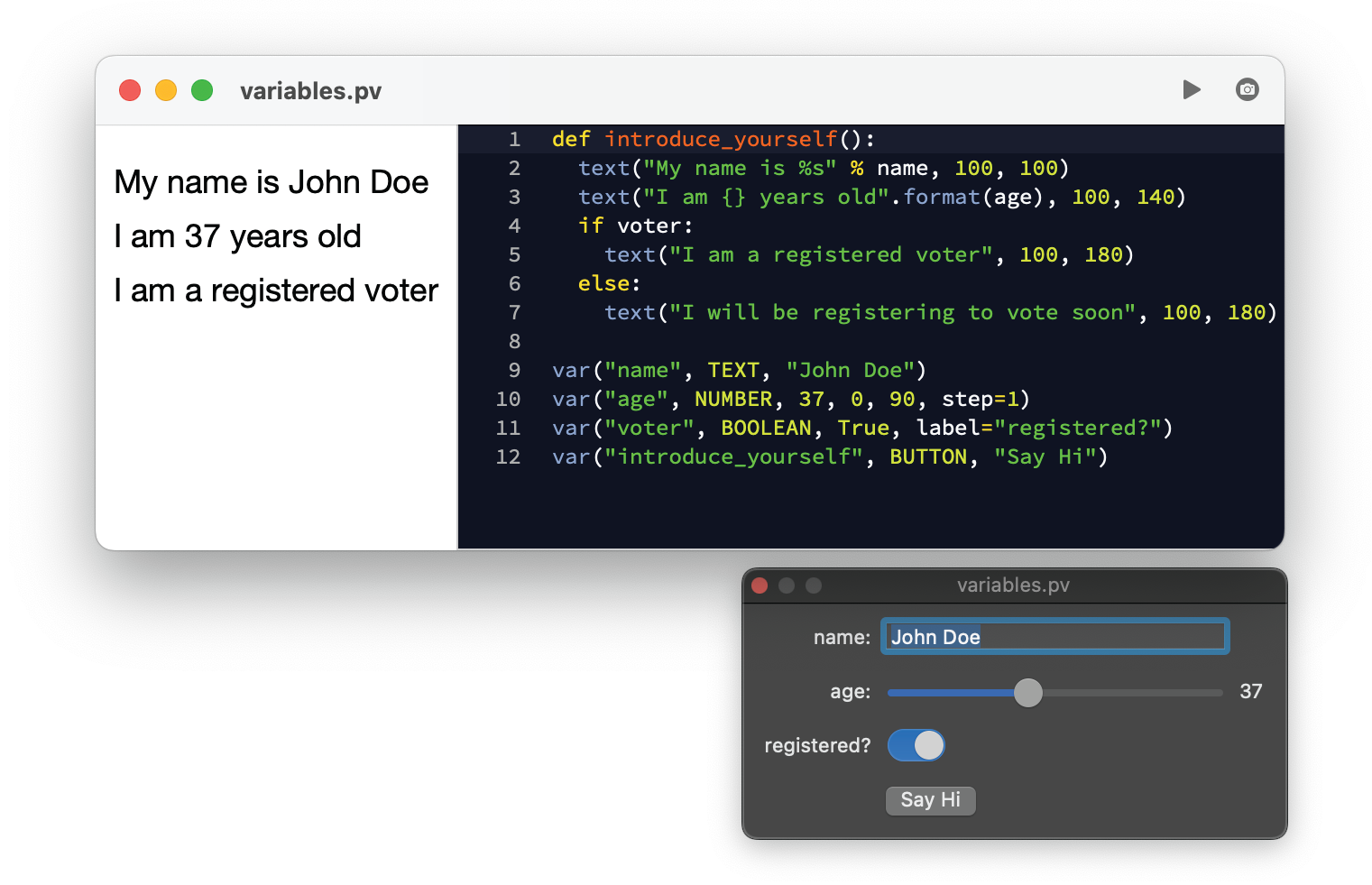
You can add user-controlled variables to the panel with the var() command.
var("name", NUMBER, value, min, max, step)
var("name", TEXT, a_string)
var("name", BOOLEAN, true_or_false)
def do_something(): print("i did it!") var('do_something', BUTTON, button_text)
You can then use the name variable in your script like any other variable. Numbers produce a slider, text an input field and booleans (True or False) a checkbox. The name parameter for buttons doesn’t actually create a variable—instead you use it to identify a function that you’ve defined in your script that should be called when the button is clicked. The button will have the name of your function as its text label unless you pass an alternate string for it to use as the third argument.
By default, the variables panel will label each of your controls using the same name as your variable, but if you provide
a label argument, you can customize it. The BUTTON
type also allows you to specify a color
value for it to use as its background:
var("surname", TEXT, "Richelieu", label="Last Name") var("search_geneology", BUTTON, "Search", label="Find Relatives", color="#f00")
The variable panel is modeled on Bob Ippolito’s widget drawer in the original DrawBot.