PlotDevice is designed to output PDF documents, images, and movies. In general it makes the trade-off of output quality over realtime responsiveness. If you’re looking to make something with low-latency interactivity (or other general whizzy-ness) you’re probably better off with a tool like NodeBox for OpenGL or Processing.
However, if you do want to experiment with interactivity in PlotDevice, there’s some built-in
support that might be of help to you. This assumes you already know how to make an animation in PlotDevice.
Interacting with the mouse
In a PlotDevice animation, there are three predefined variables that store the current position of the mouse cursor and the state of the mouse button:
MOUSEX
: the horizontal location of the mouse cursorMOUSEY
: the vertical location of the mouse cursormousedown
: is True when the mouse button is pressed, False otherwise
You would typically use the mousedown
variable in an if-statement:
if mousedown: # do stuff when the mouse is pressed else: # do stuff when the mouse isn't pressed
Interacting with the keyboard
Three predefined variables store the keys a user is pressing during a PlotDevice animation:
key
: the last key pressedkeycode
: the integer keycode of the last key pressedkeydown
: is True when a key is being pressed, False otherwise
The key
and keycode
variables are updated as long as any key is being pressed.
KEY_UP
, KEY_DOWN
, KEY_LEFT
, KEY_RIGHT
contain the keycodes for the arrow keys and the delete key is represented by KEY_BACKSPACE
.
Example
A fun example is this little sketch application. It builds up a list of points you’ve clicked-and-dragged over then draws a smooth bezier path connecting the vertices at every frame.
size(400 ,400) speed(30) def setup(anim): anim.path = [] def draw(anim): nofill() stroke(0) if mousedown: pt = Point(MOUSEX, MOUSEY) anim.path.append(pt) if anim.path: bezier(anim.path, smooth=True)
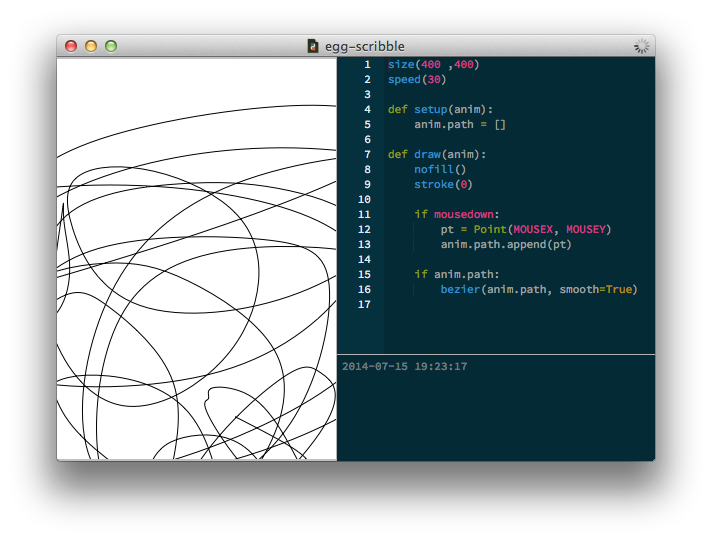