Commands
alpha()
Syntax
alpha(opacity)
Description
Sets the default opacity for all subsequent drawing operations. The new opacity value becomes part of the global state and can be reset to the default by calling alpha(1.0)
.
While other transparency effects can be achieved by setting the stroke() or fill() color to a non-opaque value, the alpha() command affects the translucency of the object as if it were a layer in Illustrator (rather than affecting the stroke and fill independently).
Context Manager
When used as part of a with
statement, alpha() treats all the drawing that occurs within the block as a single layer. As a result, overlapping objects will be flattened before the translucency is applied.
Tutorial
Example
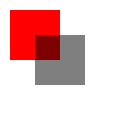
rect(10,10, 50,50, fill='red') # at full opacity alpha(.5) rect(35,35, 50,50, fill='black') # half opacity
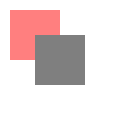
with alpha(.5): # entire layer at half opacity rect(10,10, 50,50, fill='red') rect(35,35, 50,50, fill='black')
blend()
Syntax
blend(mode)
Description
Blending operations affect how ‘old’ content on the canvas shows through new content drawn on top of it. By default the canvas uses the traditional ‘painter’s algorithm’ in which each new drawing operation obscures anything below it in proportion to its opacity.
Setting an alternate blend mode changes the rules for determining how colors combine. Different modes will use color, opacity, depth-ordering, or a combination of attributes. Call blend() with a mode-name string to set a custom blend mode.
Context Manager
When used as part of a with
statement, blend() will first render the drawing within the indented block as a single layer (using the normal
blend mode). The layer’s contents are then composited to the canvas using the specified mode.
Tutorial
Example
To demonstrate the different available modes, we’ll repeatedly draw the same foreground and backdrop images atop one another, changing only the blend mode. Note that the current alpha() level will also affect your results. Here’s a pair of test images drawn at full opacity.
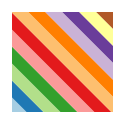
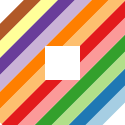
Call blend() with one of the following strings as an argument to achieve the desired compositing effect:
image("backdrop.png") blend("…") image("foreground.png")
clip()
Syntax
with clip(stencil, channel="alpha"):
Description
Sets the clipping region for a block of drawing commands.
All drawing operations within the block will be constrained by a Bezier, Image, or Text object passed as the stencil
argument. If clipping to a path, only content within the filled bounds of the stencil path will be rendered (strokes will be ignored). When clipping to an image, drawing operations will have high opacity in places where the mask value is also high.
The optional channel
arg applies only to image-based stencils and specifies which component of the stencil’s pixels should be used for this calculation. If omitted it defaults to "alpha"
(if available) or "black"
level (if the image is opaque).
When an object is used as a stencil
arg, it is ‘consumed’ by the operation and will not be directly visible on the canvas. As a result, it’s perfectly safe to include calls to Primitives or the image() command in the arguments list for clip().
See Also
The mask() command uses the same syntax as clip() but has an opposite effect.
Example
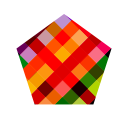
with clip(poly(64,64, 50, sides=5)): image('plaid.png')
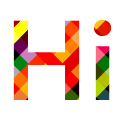
font("Avenir", "bold", 112) with clip(text('Hi', 5, 100)): image('plaid.png')
mask()
Syntax
with mask(stencil, channel="alpha"):
Description
Sets an inverted clipping region for a block of drawing commands.
All drawing operations within the block will be constrained by a Bezier, Image, or Text object passed as the stencil
argument. If masking to a path, only content drawn outside of the filled path will be visible. When masking to an image, drawing operations will have high opacity in places where the mask value is low.
The optional channel
arg applies only to image-based stencils and specifies which component of the stencil’s pixels should be used for this calculation. If omitted it defaults to "alpha"
(if available) or "black"
level (if the image is opaque).
When an object is used as a stencil
arg, it is ‘consumed’ by the operation and will not be directly visible on the canvas. As a result, it’s perfectly safe to include calls to Primitives or the image() command in the arguments list for mask().
See Also
The clip() command uses the same syntax as mask() but has an opposite effect.
Example
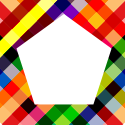
with mask(poly(64,64, 50, sides=5)): image('plaid.png')
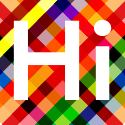
font("Avenir", "bold", 112) with mask(text('Hi', 5, 100)): image('plaid.png')
shadow()
Syntax
shadow(color, blur=10, offset=(5,5))
Description
Sets a global drop-shadow style for drawing primitives. The color
argument can be a single value, or a tuple containing valid args for the color() command. The optional blur
argument controls the sharpness of the shadowed region.
The offset
arg, if specified, sets the relative position of the shadow. It can be either a single number (with positive values corresponding to down/right and negative to up/left) or a 2-tuple with separate x & y offsets. If the offset is omitted, it will default to the same value as the blur
.
Calling shadow() affects the global state. Any subsquent drawing operations will include a drop-shadow until you call noshadow() or shadow(None)
to disable the effect.
Context Manager
When used as part of a with
statement, the shadow() command’s effects will be limited to drawing commands within the block. In addition, the shadow will be applied to drawing operations as a ‘layer’ rather than to the individual graphics objects.
Tutorial
Example
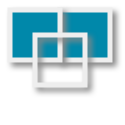
# draw each rect with its own dropshadow pen(5, fill='#08a', stroke='#eee') shadow('#999', blur=6, offset=4) rect(60,10, 50,50) rect(10,10, 50,50) rect(35,35, 50,50, fill=None)
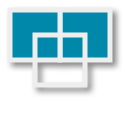
# draw a single dropshadow for the entire layer pen(5, fill='#08a', stroke='#eee') with shadow('#999', blur=6, offset=4): rect(60,10, 50,50) rect(10,10, 50,50) rect(35,35, 50,50, fill=None)
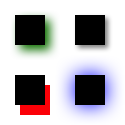
with shadow('green'): poly(30,30, 15) with shadow(('black',.5), blur=5): poly(90,30, 15) with shadow('red', blur=0, offset=(5,10)): poly(30,90, 15) with shadow('blue', blur=20, offset=0): poly(90,90, 15)
Legacy Commands
beginclip()
Syntax
beginclip(path)
Description
Using the beginclip() and endclip() commands define a clipping mask. The supplied parameter defines the path to be used as a clipping mask. Each of the shape commands returns a path that can be used with beginclip() - setting the draw parameter of a shape command simply returns the path without actually drawing the shape. Any shapes, paths, texts and images between beginclip() and endclip() are clipped, this means that any part that falls outside the clipping mask path is not drawn.
Returns
PlotDevice Equivalent
The beginclip() command has been subsumed into the new clip() command. Rather than calling beginclip() and endclip() to constrain the drawable area, you can call clip() as part of a with
statement.
Example
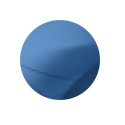
p = oval(20, 20, 80, 80, plot=False) beginclip(p) image("header.jpg", -130, 0) endclip()
endclip()
Syntax
endclip()
Description
The endclip() command is the companion to beginclip() and may only be called after the latter. When endclip() is called, subsequent shapes, paths, texts and images are no longer clipped to the path supplied to beginclip().
noshadow()
Syntax
noshadow()
Description
Clears any previously defined shadow() effects. This command is a shorthand equivalent to calling shadow(None)
.